Using the attached Eclipse Project, InterfaceAndPolymorphismAssignment.zip Download
Using the attached Eclipse Project, InterfaceAndPolymorphismAssignment.zip Download InterfaceAndPolymorphismAssignment.zip, add four more shape classes, Pyramid4 a 4-sided pyramid, Pyramid3 a 3-sided pyramid, Square, and Cube. You may add more shapes than these if you would like.
Each new shape class will implement the ShapesInterface.
You will then need to update the ShapeGenerator class to properly generate new objects of these classes.
Note that the TestDriver class never creates variables of the shape classes. It is using variables of the ShapesInteface class to access the methods of the shape objects.
Once you have made the necessary changes export your Eclipse project to a zip file and submit it to this page.
ShapeGenerator.java
/**
*
*/
import interfacePackage.ShapesInterface;
import objectsPackage.Cone;
import objectsPackage.Triangle;
import java.util.Scanner;
/**
* @author Larry Shannon
*
*/
public class ShapeGenerator
{
private static Scanner keyboard = new Scanner(System.in);
public static ShapesInterface getShape()
{
int numberOfShapes = 2; //Change this value to the number of current shapes as you add new shapes.
int randomValue = (int)(Math.random() * numberOfShapes);
ShapesInterface newShape = null;
switch(randomValue)
{
case 0:
newShape = new Triangle();
break;
case 1:
newShape = new Cone();
break;
// case 2:
// newShape = new Pyramid4();
// break;
// case 3:
// newShape = new Pyramid3();
// break;
// case 4:
// newShape = new Square();
// break;
// case 5:
// newShape = new Cube();
// break;
}
return newShape;
}
public static ShapesInterface selectShape()
{
ShapesInterface newShape = null;
boolean shapeNotSelected = true;
int menuValue = 0;
double height = 0.0;
double base = 0.0;
double radius = 0.0;
do
{
shapeNotSelected = false;
System.out.println("Select from the following shapes:");
System.out.println("1) Triangle");
System.out.println("2) Cone");
if(!keyboard.hasNextInt())
{
keyboard.nextLine();
menuValue = 0;
}
else
{
menuValue = keyboard.nextInt();
keyboard.nextLine();
}
switch(menuValue)
{
case 1:
System.out.println("You selected a Triangle.");
System.out.println("Please enter the length of the base.");
while(!keyboard.hasNextDouble())
{
keyboard.nextLine();
System.out.println("Invalid entry.");;
System.out.println("Please enter the length of the base.");
}
base = keyboard.nextDouble();
System.out.println("Please enter the height of the triangle.");
while(!keyboard.hasNextDouble())
{
keyboard.nextLine();
System.out.println("Invalid entry.");;
System.out.println("Please enter the height of the triangle.");
}
height = keyboard.nextDouble();
keyboard.nextLine();
newShape = new Triangle(height, base);
break;
case 2:
System.out.println("You selected a cone.");
System.out.println("Please enter the radius of the base.");
while(!keyboard.hasNextDouble())
{
keyboard.nextLine();
System.out.println("Invalid entry.");;
System.out.println("Please enter the radius of the base.");
}
radius = keyboard.nextDouble();
System.out.println("Please enter the height of the cone.");
while(!keyboard.hasNextDouble())
{
keyboard.nextLine();
System.out.println("Invalid entry.");;
System.out.println("Please enter the height of the cone.");
}
height = keyboard.nextDouble();
keyboard.nextLine();
newShape = new Cone(height, radius);
break;
// case 3:
// newShape = new Pyramid4();
// break;
// case 4:
// newShape = new Pyramid3();
// break;
// case 5:
// newShape = new Square();
// break;
// case 6:
// newShape = new Cube();
// break;
default:
shapeNotSelected = true;
}
}while(shapeNotSelected);
return newShape;
}
}
TestDriver.java
import java.util.ArrayList;
import java.util.Scanner;
import interfacePackage.ShapesInterface;
/**
*
*/
/**
* @author Larry Shannon
*
*/
public class TestDriver
{
private static Scanner keyboard = new Scanner(System.in);
/**
* @param args
*/
public static void main(String[] args)
{
ArrayList<ShapesInterface> myShapes = new ArrayList<ShapesInterface>();
String yesOrNo = "yes";
int arrayCount = 0;
do
{
addNewShape(myShapes);
System.out.printf("There are %d shapes in the array%n", myShapes.size());
arrayCount = 0;
for(ShapesInterface currentShape: myShapes)
{
System.out.printf("Shape #%d is a %s%n",++arrayCount, currentShape.toString());
System.out.printf("Its area is %3.2f square units%n",currentShape.getArea());
System.out.printf("Its volume is %3.2f cubic units%n",currentShape.getVolume());
currentShape.displayArea();
currentShape.displayVolume();
}
System.out.println("Would you like to add another shape?");
do
{
System.out.println("Please enter \"yes\" or \"no\"");
yesOrNo = keyboard.nextLine();
}while(!yesOrNo.equalsIgnoreCase("yes")&&!yesOrNo.equalsIgnoreCase("no"));
}while(yesOrNo.equalsIgnoreCase("yes"));
}
private static void addNewShape(ArrayList<ShapesInterface> pShapes)
{
String randomOrSelected = "r";
System.out.println("Would you like to generate a random shape or select a shape and a size?");
do
{
System.out.println("Please enter \"r\" for random or \"s\" to select the shape");
randomOrSelected = keyboard.nextLine();
}while(!randomOrSelected.equalsIgnoreCase("r")&&!randomOrSelected.equalsIgnoreCase("s"));
if(randomOrSelected.equalsIgnoreCase("r"))
{
pShapes.add(ShapeGenerator.getShape());
}
else
{
pShapes.add(ShapeGenerator.selectShape());
}
}
}
ShapeInterface.java
package interfacePackage;
/**
*
*/
/**
* @author Larry Shannon
*
*/
public interface ShapesInterface
{
public double getArea();
// This method returns the area of a 2 dimensional shape
// or the surface are of a 3 dimensional shape.
public double getVolume();
// This method returns the volume of a 3 dimensional shape
// or 0 for a 2 dimensional shape.
public void displayArea();
// This method displays the name of the shape and its area,
// If the shape is 2 dimensional it displays the 2 dimensional area
// If the shape is 3 dimensional it displays the surface area of the shape
public void displayVolume();
// This method displays the name of the shape and its volume,
// This method displays the volume of a 3 dimensional shape and 0 for a 2 dimensional shape.
}
Cone.java
/**
*
*/
package objectsPackage;
import interfacePackage.ShapesInterface;
/**
* @author Larry Shannon
*
*/
public class Cone implements ShapesInterface
{
private final double RADIUS;
private final double HEIGHT;
private final double SLANT_LENGTH;
private final double SURFACE_AREA;
private final double VOLUME;
private final String SHAPE = "Cone";
private final double PI = Math.PI;
private final double BASE_AREA;
private final double CONE_AREA;
public Cone()
{
this.RADIUS = Math.random() * 10;
this.HEIGHT = Math.random() * 10;
this.SLANT_LENGTH = calculateSlantLength();
this.CONE_AREA = calculateConeArea();
this.BASE_AREA = calculateBaseArea();
this.SURFACE_AREA = calculateSurfaceArea();
this.VOLUME = calculateVolume();
}
public Cone(double pHeight, double pRadius)
{
this.RADIUS = pRadius;
this.HEIGHT = pHeight;
this.SLANT_LENGTH = calculateSlantLength();
this.CONE_AREA = calculateConeArea();
this.BASE_AREA = calculateBaseArea();
this.SURFACE_AREA = calculateSurfaceArea();
this.VOLUME = calculateVolume();
}
private double calculateSlantLength()
{
return Math.sqrt(RADIUS * RADIUS + HEIGHT * HEIGHT);
}
private double calculateConeArea()
{
return PI * RADIUS * SLANT_LENGTH;
}
private double calculateBaseArea()
{
return PI * RADIUS * RADIUS;
}
private double calculateSurfaceArea()
{
return this.BASE_AREA + this.CONE_AREA;
}
private double calculateVolume()
{
return (BASE_AREA * HEIGHT) / 3;
}
@Override
public double getArea()
{
return this.SURFACE_AREA;
}
@Override
public double getVolume()
{
return this.VOLUME;
}
@Override
public void displayArea()
{
System.out.printf("This is a %s with an area of %3.2f square units%n",SHAPE, SURFACE_AREA);
}
@Override
public void displayVolume()
{
System.out.printf("This is a %s with a volume of %3.2f cubic units%n",SHAPE, VOLUME);
}
public String toString()
{
return SHAPE;
}
}
Triangle.java
/**
*
*/
package objectsPackage;
import interfacePackage.ShapesInterface;
/**
* @author Larry Shannon
*
*/
public class Triangle implements ShapesInterface
{
private final double BASE;
private final double HEIGHT;
private final double SURFACE_AREA;
private final String SHAPE = "Triangle";
public Triangle()
{
this.BASE = Math.random() * 10;
this.HEIGHT = Math.random() * 10;
this.SURFACE_AREA = (this.BASE * this.HEIGHT) / 2;
}
public Triangle(double pHeight, double pBase)
{
this.BASE = pBase;
this.HEIGHT = pHeight;
this.SURFACE_AREA = calculateSurfaceArea();
}
private double calculateSurfaceArea()
{
return (this.BASE * this.HEIGHT) / 2;
}
@Override
public double getArea()
{
// TO DO .Auto-generated method stub
return this.SURFACE_AREA;
}
@Override
public double getVolume()
{
// A triangle does not have a volume
return 0;
}
@Override
public void displayArea()
{
System.out.printf("This is a %s with an area of %3.2f square units%n",SHAPE, SURFACE_AREA);
}
@Override
public void displayVolume()
{
System.out.printf("This is a %s and it has no volume!%n",SHAPE);
}
public String toString()
{
return SHAPE;
}
}
Best Answer
These are the modifications I made. Please review this and let me know if you have any problems running it or if you have any questions about it. Please read the notes below as well. Thank you.
答案中图片缺失,下载完整PDF文档,请至https://studyokk.com/doc-view-226html购买下载
ShapeGenerator.java
import interfacePackage.ShapesInterface; import objectsPackage.Cone; import objectsPackage.Cube; import objectsPackage.Pyramid3; import objectsPackage.Pyramid4; import objectsPackage.Square; import objectsPackage.Triangle; import java.util.Scanner; /** * @author Larry Shannon * */ public class ShapeGenerator { private static Scanner keyboard = new Scanner(System.in); public static ShapesInterface getShape() {此内容售价The price for this content is ¥5,请点击购买按钮使用支付宝进行支付! Please pay with Alipay to view the full content!购买内容 Unlock full access
While the parameterized constructor will make use of the given parameters in initializing these fields.
public Pyramid3(double height, double base, double slant_height) { HEIGHT = height; BASE = base; SLANT_HEIGHT = slant_height; SURFACE_AREA = getArea(); VOLUME = getVolume(); }
In this class, we will also define and provide the method "calculateBaseArea" that calculates the base area of the pyramid. Now that the base is triangle and not sqaure, we will use the formula for the area of the triangle to get the area of the base of this pyramid.
private double calculateBaseArea() { return (1.0/2) * BASE * HEIGHT; }
And after that, we will directly implement the getArea() function which will use the formula BA + 3/2 * h * s where "BA" is the area of the base which was calculated by the calculateBaseArea() method, "h" is the height and "s" is the slant height.
public double getArea() { return calculateBaseArea() + ((3.0 / 2) * HEIGHT * SLANT_HEIGHT); }
To get this pyramids volume, we will follow the formula √3 / 12 * b2 * h where we will first get the square root of 3 and divide it by 12. The result will then be multiplied to the height and base squared.
public double getVolume() { return (Math.sqrt(3) / 12) * (BASE * BASE) * HEIGHT; }
Step 4: Define the Square class.
This next class that we will define will model a 2-dimensional shape, square. Since a square is a rectangular shape that has the same length of sides, this class will have a fields for the length of the side.
public class Square implements ShapesInterface { private final double SIDE_LENGTH; private final double SURFACE_AREA; private final String SHAPE = "Square";
The default constructor will randomize the side length between 0 and 10 and the parameterized constructor will make use of the given parameter to initialize the side length.
public Square() { SIDE_LENGTH = Math.random() * 10; SURFACE_AREA = getArea(); } public Square(double side_length) { SIDE_LENGTH = side_length; SURFACE_AREA = getArea(); }
For its implementation of getArea(), the function will simply calculate the area of a square by squaring the length of the side. This means that the function will return the SIDE_LENGTH multiplied to itself.
public double getArea() { return SIDE_LENGTH * SIDE_LENGTH; }
It will have an implementation of the getVolume() function but we will let it return 0 since a square has no volume as it is only a 2D shape.
public double getVolume() { return 0; }
This means that the displayVolume() will notify the user that this shape has no volume as well.
public void displayVolume() { System.out.printf("This is a %s and it has no volume!%n",SHAPE); }
Step 5: Define the Cube class.
The last class that we will define is the Cube class which will be a 3-dimensional shape representation of the square. With this, it will also have a field for the length of the sides of a cube.
public class Cube implements ShapesInterface { private final double SIDE_LENGTH; private final double SURFACE_AREA; private final double VOLUME; private final String SHAPE = "Cube";
To calculate its area in getArea() function, we will simply get the area of one of its faces (since a face is also a square, we will use square's formula) by multiplyign the SIDE_LENGTH by itself.
(SIDE_LENGTH * SIDE_LENGTH)
After that, we will multiply this face area by 6 since there are six faces of a cube. The result will then be the area of a cube.
public double getArea() { return 6 * (SIDE_LENGTH * SIDE_LENGTH); }
To calculate its volume, we will simply follow the formula s3 where it needs the side length to be multiplied to itself twice.
public double getVolume() { return SIDE_LENGTH * SIDE_LENGTH * SIDE_LENGTH; }
Sample output: This is a sample run of the program.

Image transcription text
Console X 1+ import java. util. ArrayList;_ <terminated> TestDriver [Java
Application] C:\Program Files\Java\jdk-18.0.2\bin\javaw.exe (14 Apr...

Image transcription text
) Square 18 * / 6) Cube > D Cube.java 190 public static void main (String arg
5 > Pyramid3.java 20 ArrayList <ShapesInterface> mySha Yo...
Key references:
Surface Area of a Square Pyramid - Formula, Examples, Definition. (2023). Retrieved 14 April 2023, from https://www.cuemath.com/measurement/surface-area-of-square-pyramid/
Surface Area of Triangular Pyramid - Formula, Examples, Definition. (2023). Retrieved 14 April 2023, from https://www.cuemath.com/measurement/surface-area-of-triangular-pyramid-formula/
Surface Area of Cube (Formula & Solved Examples). (2023). Retrieved 14 April 2023, from https://byjus.com/maths/surface-area-of-cube/
Volume of a Square Pyramid Formula - GeeksforGeeks. (2022). Retrieved 14 April 2023, from https://www.geeksforgeeks.org/volume-of-a-square-pyramid-formula/
Pyramid Volume Calculator. (2023). Retrieved 14 April 2023, from https://www.omnicalculator.com/math/pyramid-volume
Volume of a Cube Calculator | Formula. (2023). Retrieved 14 April 2023, from https://www.omnicalculator.com/math/cube-volume
Contact Us
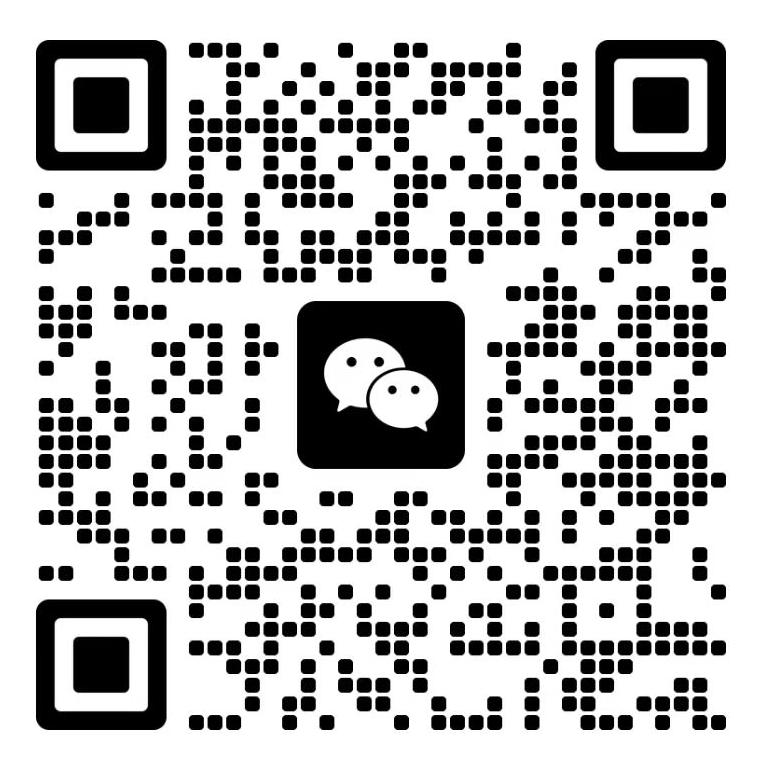
扫码联系微信客服 享一对一做题服务
猜你喜欢
- Customized Homework Help2023-11-02
- Discuss Tesla ERRC and their canvas in detail and include why you think they have Blue Ocean Strateg2023-11-01
- In the regular Stable Match- ing problem, we assumed that participants prefer to be matched over bei2023-10-29
- Failed RECAPTCHA check.是怎么回事?2023-10-29
- Calculate the profit or loss from the position in the futures market if in 3 months, the contracts a2023-10-27
- Which of the following best describes the risks associated with futures contracts?2023-10-27
- Suppose that the methods of this problem are used to forecast a value of Y for a combination of Xs v2023-10-25
- The Board of Directors would like you to undertake the following tasks: 2023-10-13
- Explain the term discontinuous innovation and provide an example.2023-10-12
- produce a 3000-word report critically considering, in context of Bandon2023-10-07
Post Reply